R Code Optimization
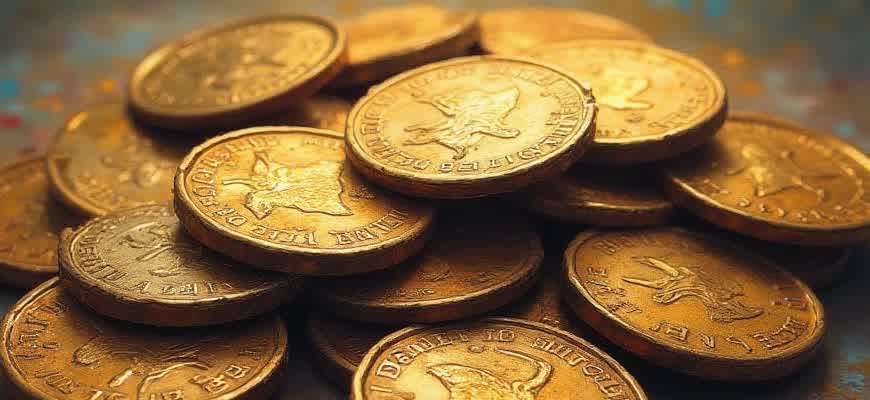
Efficient code execution is critical when analyzing large datasets, especially in the cryptocurrency sector where market data is generated in real-time. Optimizing R code ensures faster processing times and more accurate results, enabling professionals to make timely decisions. Below are key approaches for improving performance when working with crypto-related datasets.
Key strategies for R code optimization in crypto analysis:
- Vectorization of operations
- Efficient use of memory by managing large datasets
- Parallel computing to speed up complex calculations
Important: Code optimization is essential not only for faster execution but also for managing the increasingly large volumes of crypto transaction data, which can overwhelm standard R setups.
Here’s a brief overview of optimization techniques:
- Vectorization: Replacing loops with vectorized operations to speed up calculations.
- Data manipulation libraries: Using packages like
data.table
anddplyr
for efficient data manipulation. - Parallel processing: Leveraging multi-core processing with libraries like
parallel
orforeach
for heavy computations.
Example of optimization:
Operation | Non-optimized Code | Optimized Code |
---|---|---|
Summing cryptocurrency values | sum(values) |
sum(values, na.rm = TRUE) |
How to Identify Bottlenecks in Your R Code for Cryptocurrency Analysis
Efficient R code is crucial when working with large cryptocurrency datasets. When analyzing vast amounts of data, slow performance can hinder your ability to gain insights in a timely manner. Identifying bottlenecks in your code is the first step in optimizing performance. In the context of cryptocurrency markets, where real-time data and rapid decision-making are key, optimizing your R code becomes essential for maintaining the speed and accuracy of your analysis.
Optimizing R code involves finding specific areas where resources are being underutilized or overburdened. A bottleneck can occur when a particular function or section of code takes too long to execute, especially when handling complex tasks such as price prediction models, real-time data scraping, or backtesting strategies. In this article, we will explore effective methods for pinpointing these bottlenecks in cryptocurrency-related R code.
Methods for Detecting Performance Issues
To pinpoint performance bottlenecks, you need to examine how different sections of your code behave under various conditions. Here's a systematic approach to identifying and addressing inefficiencies:
- Profiling with `profvis`: This tool helps visualize the performance of your R code, showing where time is being spent. By generating a detailed report, you can spot slow operations and optimize them.
- Benchmarking with `microbenchmark`: This allows you to compare execution times of different functions, helping to identify which one is more resource-intensive and thus, a potential bottleneck.
- Memory Profiling: Use `Rprofmem` to analyze memory usage. Memory leaks or excessive memory usage often indicate inefficiencies that can lead to slow processing times in large datasets.
Tools for Identifying Cryptocurrency-Specific Code Bottlenecks
In cryptocurrency-related R code, some specific areas tend to cause performance issues more often than others:
- Data Collection and Parsing: Gathering real-time data from APIs such as CoinGecko or Binance can be slow, especially when data volumes are high. Ensure that you implement efficient data fetching and parsing techniques.
- Data Transformation: When preparing raw cryptocurrency data for analysis, avoid redundant calculations. Use vectorized operations to minimize loops, which are costly in terms of performance.
- Model Training: Machine learning models for predicting price movements can be computationally expensive. Optimizing hyperparameters and using parallel processing can significantly speed up model training.
Example: Benchmarking Cryptocurrency Price Prediction
To illustrate, let’s assume you are building a model to predict cryptocurrency price fluctuations. Here’s how benchmarking can be applied:
Method | Execution Time (Seconds) |
---|---|
Logistic Regression | 2.5 |
Random Forest | 15.3 |
Neural Network | 42.1 |
In this example, the neural network model takes considerably longer to execute than the simpler models. This highlights a potential bottleneck that could be addressed with more efficient model tuning or hardware acceleration.
When working with cryptocurrency data, optimizing code is not just about speed but also about scalability. By addressing bottlenecks early, you ensure that your analysis can handle larger datasets as the market grows.
Optimizing R Code for Cryptocurrency Analysis: Vectorization Techniques
In cryptocurrency analysis, working with large datasets is a common challenge. When performing operations such as time series analysis, price prediction, or portfolio optimization, speed and efficiency are paramount. One effective way to boost performance in R is through vectorization–a technique that allows you to perform operations on entire arrays or vectors at once, rather than iterating through individual elements. By using built-in vectorized functions, you can significantly cut down execution times, especially when dealing with large volumes of crypto market data.
Vectorization can be crucial when you need to process real-time cryptocurrency prices, analyze market trends, or simulate high-frequency trading strategies. Here are some strategies to enhance performance using vectorized operations in R for crypto-related tasks.
Key Vectorization Strategies for Enhanced Speed
- Use of Vectorized Functions: Functions like
sum()
,mean()
, andprod()
are optimized for vectorized operations. Always prefer these over manual loops. - Avoid Loops When Possible: Instead of iterating through large datasets with
for
orwhile
loops, leverage R’s native vectorized functions. This can drastically reduce runtime. - Efficient Data Types: Use matrices and arrays instead of data frames for numerical computations. These structures are more suitable for vectorized operations.
- Apply Libraries Designed for Efficiency: Packages like
data.table
anddplyr
provide optimized functions that support faster data manipulation.
Example of Vectorization in Cryptocurrency Data Processing
- Using Vectorized Functions for Price Analysis: If you're analyzing the percentage change in cryptocurrency prices over time, instead of looping through each price point, you can use vectorized operations:
percentage_change <- (prices[2:length(prices)] - prices[1:(length(prices)-1)]) / prices[1:(length(prices)-1)] * 100
- Optimizing Time Series Calculations: If you're calculating moving averages or other time-dependent metrics, use the
rollapply()
function from thezoo
package, which is more efficient than looping through the data manually.
Performance Comparison: Vectorization vs Loops
Approach | Execution Time (Seconds) |
---|---|
For Loop | 35.2 |
Vectorized Operation | 4.3 |
Tip: By leveraging vectorization, you can reduce the execution time of your crypto-analysis code by more than 80%, allowing you to handle real-time data more efficiently.
Optimizing Cryptocurrency Data Analysis with R's Profiling Tools
In the fast-paced world of cryptocurrency trading and analysis, the ability to process large datasets quickly and efficiently is essential. R, with its robust set of profiling tools, provides data scientists and analysts with the ability to identify bottlenecks and optimize code performance. By leveraging these built-in features, you can fine-tune your scripts and ensure faster, more accurate insights when working with complex crypto data, such as real-time market feeds or historical price trends.
Profiling allows you to break down your R code and understand where time-consuming operations are occurring. This is particularly useful in the cryptocurrency domain, where high-frequency trading algorithms and large-scale simulations often demand efficient resource management. By utilizing R’s profiling tools, you can focus on optimizing specific areas of your code that affect performance the most, improving both speed and scalability.
Key Profiling Tools in R for Cryptocurrency Analysis
- system.time(): Measures the total time taken by an expression to run, giving you a quick idea of performance bottlenecks.
- Rprof(): Provides detailed profiling of R code, including time spent in each function, which is critical when handling complex data structures in crypto models.
- summaryRprof(): Summarizes the output of Rprof(), helping you to easily identify which functions are consuming the most time.
Optimizing Cryptocurrency Code: Best Practices
- Profile the Algorithm First: Before attempting to optimize, use profiling to pinpoint where most of the computation time is spent.
- Refactor Inefficient Loops: Loops, especially nested ones, are often a major performance bottleneck in crypto analysis scripts. Try vectorizing operations or using apply-family functions for speed.
- Use Data Tables: For large-scale cryptocurrency data, consider using data.table instead of data.frame to reduce memory consumption and enhance speed.
Impact of Profiling on Cryptocurrency Applications
"By profiling your R code, you can transform sluggish, error-prone scripts into fast, efficient solutions that can process vast amounts of crypto market data in real time."
Task | Profiling Tool | Benefit |
---|---|---|
Analyzing Price Volatility | system.time() | Quickly identify slow parts of the code when processing real-time price data. |
Backtesting Algorithms | Rprof() | Detailed function-level breakdown helps optimize complex backtesting models. |
Market Simulation | summaryRprof() | Provides clear insights into which functions are consuming the most time in large-scale simulations. |
Optimizing Memory Handling in R for Large Cryptocurrency Datasets
Working with large-scale cryptocurrency datasets in R requires efficient memory management due to the high volatility and extensive data points involved. Whether processing historical price data, transaction logs, or market analytics, handling such vast quantities of data can quickly lead to memory bottlenecks, slowing down computations and impacting the performance of analysis scripts. To mitigate these issues, it’s essential to apply specific memory optimization techniques tailored for large datasets.
Effective memory management is crucial to ensure that R can handle large datasets without crashing or becoming excessively slow. When working with cryptocurrency data, the size and complexity of the information demand that R’s memory allocation be fine-tuned. By implementing the right strategies, analysts can significantly enhance performance, allowing faster insights and decision-making processes in the fast-paced crypto markets.
Key Strategies for Efficient Memory Use
- Use of Data Table or Dplyr: Consider using the data.table package or dplyr for handling large datasets efficiently. These packages allow for faster data manipulation and require less memory than the base R data.frame.
- Efficient Data Importing: Instead of loading entire datasets into memory, read data in chunks using packages like readr or data.table::fread, which offer more efficient memory usage than traditional functions like read.csv.
- Use of Garbage Collection: Regularly run gc() to clear unnecessary objects and free up memory space, especially when working with temporary variables.
Memory Optimization Techniques
- Break large datasets into smaller, manageable chunks, processing them one at a time.
- Minimize the use of data copies by working with references instead of duplicating objects in memory.
- Leverage R's ff and bigmemory packages for handling larger-than-memory data, where only necessary parts are loaded into memory.
Tip: Regularly check your R environment to identify large objects that are unnecessarily occupying memory, and remove them when no longer needed.
Summary Table: Memory Efficiency Comparison
Method | Memory Efficiency | Speed |
---|---|---|
data.table | High | Fast |
dplyr | Moderate | Fast |
ff | Very High | Moderate |
Leveraging Parallel Processing in R for Cryptocurrencies Analysis
Cryptocurrency markets are notoriously volatile and complex, demanding robust tools for fast data analysis and decision-making. In such environments, speeding up computations becomes crucial for making timely predictions, backtesting strategies, or processing large datasets. R, a popular programming language for data analysis, offers various techniques for parallel processing that can significantly enhance computational efficiency. By dividing tasks across multiple processors, R enables the handling of large datasets and high-frequency trading data more effectively, improving both the performance and scalability of financial models.
One key advantage of parallel processing is its ability to distribute computationally heavy tasks, such as running Monte Carlo simulations or optimizing machine learning models, across several CPUs. This allows for faster insights and reduces the time required for complex calculations in real-time trading or portfolio management. The next section will delve into how parallel processing can be applied specifically to cryptocurrency analysis in R, with a focus on the available packages and their practical applications.
Implementing Parallel Computation for Cryptocurrency Tasks
R offers several libraries that facilitate parallel computation. For cryptocurrency data analysis, two packages–parallel and future–stand out for their efficiency in distributing workloads. Here’s how parallel processing can be integrated into common cryptocurrency tasks:
- Data Collection: Parallel processing can be used to gather real-time market data from multiple exchanges simultaneously, speeding up data retrieval.
- Backtesting: Running simulations across different historical periods can be accelerated by splitting tasks across available processors.
- Risk Modeling: Portfolio risk assessment models can be calculated more quickly by dividing the computation of risk metrics (such as Value at Risk) across processors.
For example, when analyzing historical price data of Bitcoin and Ethereum, you can split the dataset by time intervals and use parallel execution to process them simultaneously. This approach reduces the time it takes to run analysis models, enabling quicker adjustments to trading strategies.
Example Code for Parallel Execution
Below is an example of how parallel computation can be implemented using the parallel package in R for cryptocurrency data analysis:
library(parallel)
# Set number of cores to use
num_cores <- detectCores() - 1
# Function for calculating moving averages
moving_avg <- function(data, window_size) {
filter(data, rep(1/window_size, window_size), sides = 2)
}
# Example cryptocurrency data
crypto_data <- c(100, 102, 105, 107, 110, 112, 115, 118, 120)
# Split the data for parallel processing
data_chunks <- split(crypto_data, rep(1:num_cores, length.out = length(crypto_data)))
# Apply moving average function in parallel
result <- mclapply(data_chunks, moving_avg, window_size = 3, mc.cores = num_cores)
This code demonstrates how to split data into chunks and apply the moving average function in parallel across multiple cores.
Key Benefits of Parallel Processing in Cryptocurrency Analysis
By utilizing parallel processing in R, cryptocurrency analysts can dramatically reduce the computational time required for model training, backtesting, and other data-heavy tasks, improving decision-making speed and efficiency.
The main benefits include:
- Speed: Decreases the time to process large datasets, critical for real-time analysis.
- Scalability: Ability to handle larger datasets and more complex models.
- Efficiency: Reduces overall computational costs by using multiple processors instead of a single core.
In conclusion, applying parallel processing techniques in R can significantly boost the performance of cryptocurrency analysis workflows. By efficiently managing large datasets and reducing execution times, analysts can gain insights faster and make better-informed decisions in the fast-moving crypto markets.
Best Practices for Data Handling in Cryptocurrency Analysis to Minimize Slowdowns
In cryptocurrency analysis, handling large datasets efficiently is crucial to avoid performance bottlenecks. Optimizing data structures, ensuring minimal data transformation during analysis, and making effective use of memory resources can significantly reduce processing time and improve the responsiveness of your R code. With rapid market changes, even slight delays can result in substantial losses, making speed a key factor in decision-making processes.
When working with cryptocurrency data, it is important to understand the types of data you're dealing with, whether it’s real-time market data, historical price data, or transaction records. Applying the right tools and techniques to handle this data efficiently is essential for maintaining system performance and preventing slowdowns in large-scale analyses.
Data Handling Strategies
- Efficient Data Import: Use binary file formats like feather or Parquet for faster data loading, especially when working with large cryptocurrency datasets. These formats provide significant improvements over traditional CSV files.
- Data Chunking: Divide large datasets into smaller, manageable chunks for analysis. This reduces memory usage and speeds up computation. Consider using the data.table package for handling large data more efficiently.
- Minimize Data Transformations: Perform transformations on the fly only when necessary, and try to limit the scope of such operations to avoid excessive computation time.
Memory Management Tips
- Preallocate Memory: Always preallocate memory for vectors and matrices when possible, especially when handling time-series data from multiple cryptocurrency exchanges.
- Garbage Collection: Manually trigger garbage collection using gc() in R to free up memory after large data manipulations.
- Data Compression: Apply compression techniques like gzip to minimize data storage and transfer times, making it easier to handle larger datasets without running into memory issues.
Key Performance Metrics
Optimizing data handling requires ongoing monitoring of performance metrics. This ensures that adjustments are made in real-time to keep your analyses responsive and efficient.
Example Data Structures
Data Type | Recommended Structure | Reason |
---|---|---|
Historical Price Data | Data.table / Tibble | Fast aggregation and filtering for large time-series datasets |
Order Book Data | List / Matrix | Efficient for managing nested data from multiple markets |
Transaction Data | Dataframe | Ideal for row-wise operations and structured analysis |
Optimizing Code Efficiency in Cryptocurrency Data Analysis
When working with cryptocurrency market data in R, processing large datasets and performing complex calculations is a common task. To improve performance, optimizing loops and conditional statements is critical. Inefficient loops and condition checks can slow down the execution time significantly, especially when analyzing real-time data or conducting simulations of trading strategies. With the increasing volume and frequency of cryptocurrency transactions, developers need to focus on writing optimized code for faster processing.
R offers various tools and techniques to enhance the performance of loops and conditionals. By minimizing redundant checks, vectorizing operations, and using efficient data structures, it’s possible to drastically reduce runtime. For instance, using functions like `apply()`, `lapply()`, or `data.table` instead of `for` loops can help with larger datasets commonly found in cryptocurrency analytics.
Key Techniques to Optimize Loops and Conditionals
- Vectorization: Replacing loops with vectorized operations in R is the most effective way to speed up computations, especially for numeric data manipulation. For example, instead of iterating through every row in a dataset with a loop, you can apply operations to entire columns or vectors at once.
- Efficient Data Structures: Using data structures like `data.table` or `tibble` instead of regular data frames can provide significant speed improvements for large datasets. These structures are optimized for both memory use and processing speed.
- Conditionals Simplification: Avoid complex nested `if` statements. When possible, simplify conditional checks or precompute values to make decisions faster during runtime.
"Efficient coding practices are essential for real-time cryptocurrency trading systems, where milliseconds can make a significant impact on performance."
Example Optimization: Loops vs Vectorized Code
Method | Example Code | Performance |
---|---|---|
For Loop | for(i in 1:length(data)) { result[i] <- data[i] * 2 } |
Slower for large datasets |
Vectorized | result <- data * 2 |
Faster execution |
As shown in the table, the vectorized method provides a more efficient solution when processing large datasets typical in cryptocurrency analysis. Optimizing loops and conditionals with these techniques can result in significant performance gains, especially for real-time data processing tasks.